R Language –Data Structures : Online Course
R List
- Vector is a sequence of a single data type, but a List is a sequence of one or more data types.
- A list is a collection of objects each of which can be almost anything.
- List can have data types like numeric, integer, character, complex, logical and vector. List can also contain matrices.
- The list() function is used to create a list in R.
List in R Programming
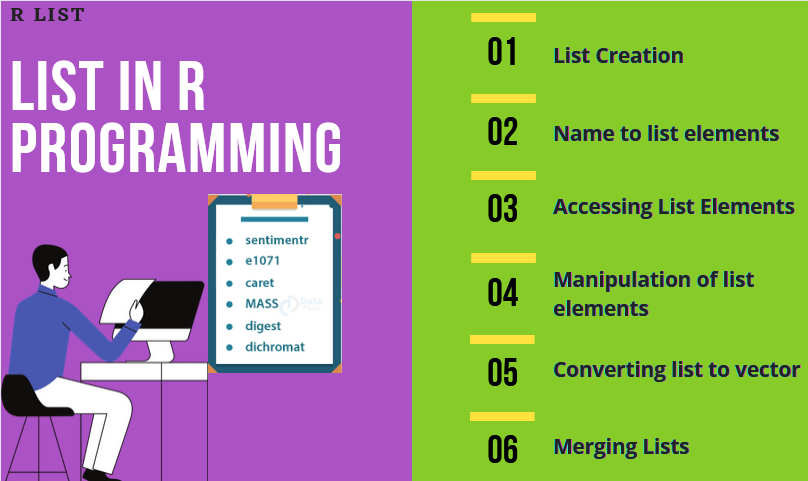
Creating List
Example to create a list containing strings, numbers, vectors and a logical values.
Input: Create a list containing strings, numbers, vectors and a logical values. |
list1 <- list(“Red”, c(21,32,11), 5L, 3+1i, TRUE, 51, 119.1) print(list1) |
Output: |
[[1]] [1] “Red” [[2]] [1] 21 32 11 [[3]] [1] 5 [[4]] [1] 3+1i [[5]] [1] TRUE [[6]] [1] 51 [[7]] [1] 119.1 |
List using Sequence in R
You can create list as a sequence of numbers.
Input: The list has been created using the sequence 6:11. |
list1 <- 6:11 print(list1) |
Output: |
[1] 6 7 8 9 10 11 |
Naming List Elements
Naming List Elements using names() in R: For example, each element of the list is named using the names() function. List elements can be given names and can be accessed using these names.
Input: Creating a list containing a vector, a matrix , complex and a list. |
l = list(5, 8, c(5, 9, 10), 2+1i, matrix(c(40,80,60,70,90,80), nrow = 2)) names(l) = c(‘Number’, ‘Number’, ‘Vector’, ‘Complex’, ‘Matrix’) l |
Output: |
$Number [1] 5 $Number [1] 8 $Vector [1] 5 9 10 $Complex [1] 2+1i $Matrix [,1] [,2] [,3] [1,] 40 60 90 [2,] 80 70 80 |
Accessing List Elements
We can also access the elements of the list by using index.
We can retrieve a list fragment with the single square bracket “[]” operator. lets see the example
Input: # Create a list containing a number, vector, a matrix and a list. |
l = list(5, 8, c(5, 9, 10), 2+1i, matrix(c(40,80,60,70,90,80), nrow = 2)) l[1] #retrieve Single member l[5] l[c(2, 3)] #retrieve multiple member |
Output: |
retrieve single member [[1]] [1] 5 [[1]] [,1] [,2] [,3] [1,] 40 60 90 [2,] 80 70 80 retrieve multiple member [[1]] [1] 8 [[2]] [1] 5 9 10 |
If you want to access the direct elements of the list, you must use the double slicing operator “[[ ]]” which is two square brackets. We can modify its content directly.
Input: Create a list containing a number, vector, a matrix and a list. |
l = list(5, 8, c(5, 9, 10), 2+1i, matrix(c(40,80,60,70,90,80), nrow = 2)) #Access the direct elements of the list l[[3]] #Modify its content directly l[[3]][1] = 8 l[[3]] |
Output: |
[1] 5 9 10 Modify its content directly [1] 8 9 10 |
Manipulating List Elements
We can add, remove and update list elements. We can only add and remove elements at the end of the list. But we can update any element. Let see the example.
Input: Create a list containing a number, vector, a matrix and a list. |
l = list(c(5, 9, 10), 2+1i, matrix(c(40,80,60,70,90,80), nrow = 2), 10) l[2] Add element at the end of the list. l[5] = 2 l Remove element at the end of the list. l[2] = NULL l Updating the 2nd Element. l[2] = ‘Hii’ l |
Output: |
[[1]] [1] 2+1i Add element at the end of the list. [[1]] [1] 5 9 10 [[2]] [1] 2+1i [[3]] [,1] [,2] [,3] [1,] 40 60 90 [2,] 80 70 80 [[4]] [1] 10 [[5]] [1] 2 Remove element at the end of the list. [[1]] [1] 5 9 10 [[2]] [,1] [,2] [,3] [1,] 40 60 90 [2,] 80 70 80 [[3]] [1] 10 [[4]] [1] 2 Updating the 2nd Element. [[1]] [1] 5 9 10 [[2]] [1] “Hii” [[3]] [1] 10 [[4]] [1] 2 |
Converting List to Vector
A list can be converted to a vector so to perform all arithmetic operations, lists must be converted to vectors using the unlist() function.
Input: For example, list has been converted into unlist or vector. |
l1 = list(c(5, 9, 10), 2+1i, matrix(c(40,80,60,70,90,80), nrow = 2)) ul = unlist(l1) ul |
Output: |
[1] 5+0i 9+0i 10+0i 2+1i 40+0i 80+0i 60+0i 70+0i 90+0i 80+0i |
Example 2: Create a list and Convert into vector
Input: Create a list and Convert into vector |
Create lists. l1 <- list(1:5) print(l1) l2 <-list(10:14) print(l2) Convert the lists to vectors. v1 <- unlist(l1) v2 <- unlist(l2) print(v1) print(v2) Now add the vectors result <- v1+v2 print(result) |
Output: |
[[1]] [1] 1 2 3 4 5 [[1]] [1] 10 11 12 13 14 [1] 1 2 3 4 5 [1] 10 11 12 13 14 [1] 11 13 15 17 19 |
Merging Lists
You can merge multiple lists into a single list by placing all the lists inside a single list() function.
Input: For example, list l1 and l2 have been combined. |
l1 = list(c(5, 9, 10), 2+1i, matrix(c(40,80,60,70,90,80), nrow = 2)) l2 = list(‘hii’, 10, 5) c(l1, l2) |
Output: |
[[1]] [1] 5 9 10 [[2]] [1] 2+1i [[3]] [,1] [,2] [,3] [1,] 40 60 90 [2,] 80 70 80 [[4]] [1] “hii” [[5]] [1] 10 [[6]] [1] 5 |
R Language: Online Course
आशा करता हूँ, कि यह आर्टिकल आपको पसंद आया होगा तो सोच क्या रहे हैं अभी इसी वक्त इसे अपने दोस्तों के साथ सोशल मीडिया पर Share करें।
Thanking You………………धन्यवाद………………..शुक्रिया………………..मेहरबानी…………………..
Read More
Reference